In WooCommerce, product prices by default are displayed as “$5.00” for simple products and “$5.00 – $20.00” for variable products. Ever wanted to add text before or after the pricing display, like this: “Only $5.00” or “$5.00 limited time only”?
There are a number of plugins that can help do this, but maybe you want to do this yourself using a small code snippet or want to add in some custom conditions to determine when the text should appear. Read on to find out how to add text before or after product prices programmatically in WooCommerce.
Table of Contents
How prices are displayed in WooCommerce
In WooCommerce, the default price display for a product is as follows, dependent on the product type:
- Simple products
- $5.00
- Variable products
- $5.00 – $20.00
The HTML of the price displayed is filterable via the woocommerce_get_price_html
filter hook.
This filter hook allows extensibility of the price display to completely overhaul the markup or to add some text before or after the price.
I’ll be using code snippets to add the additional text. Unsure how to use snippets? Check out my post here.
Adding text before the price
You can use the following code snippet to add some text before the price.
function yourprefix_product_price_before_text( $price, $product ) {
if ( !is_admin() ) { // This means it doesn't effect the price display in your dashboard, such as on the list of products
$price = 'Text before ' . $price;
}
return $price;
}
add_filter( 'woocommerce_get_price_html', 'yourprefix_product_price_before_text', 10, 2 );
Pricing will then display like this on product pages, categories, etc:
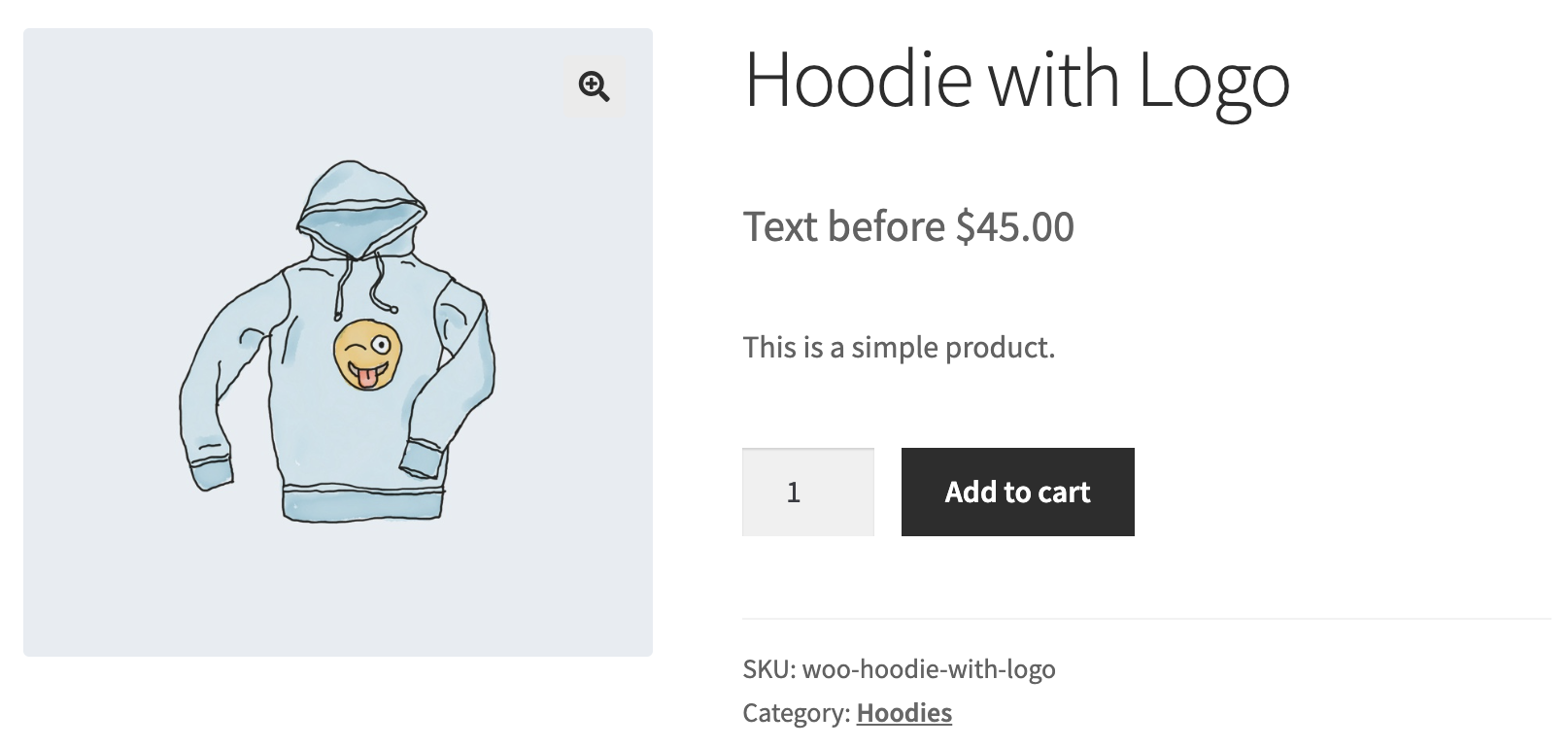
Adding text after the price
You can use the following code snippet to add some text after the price.
function yourprefix_product_price_after_text( $price, $product ) {
if ( !is_admin() ) { // This means it doesn't effect the price display in your dashboard, such as on the list of products
$price = $price . ' Text after';
}
return $price;
}
add_filter( 'woocommerce_get_price_html', 'yourprefix_product_price_after_text', 10, 2 );
Pricing will then display like this on product pages, categories, etc:
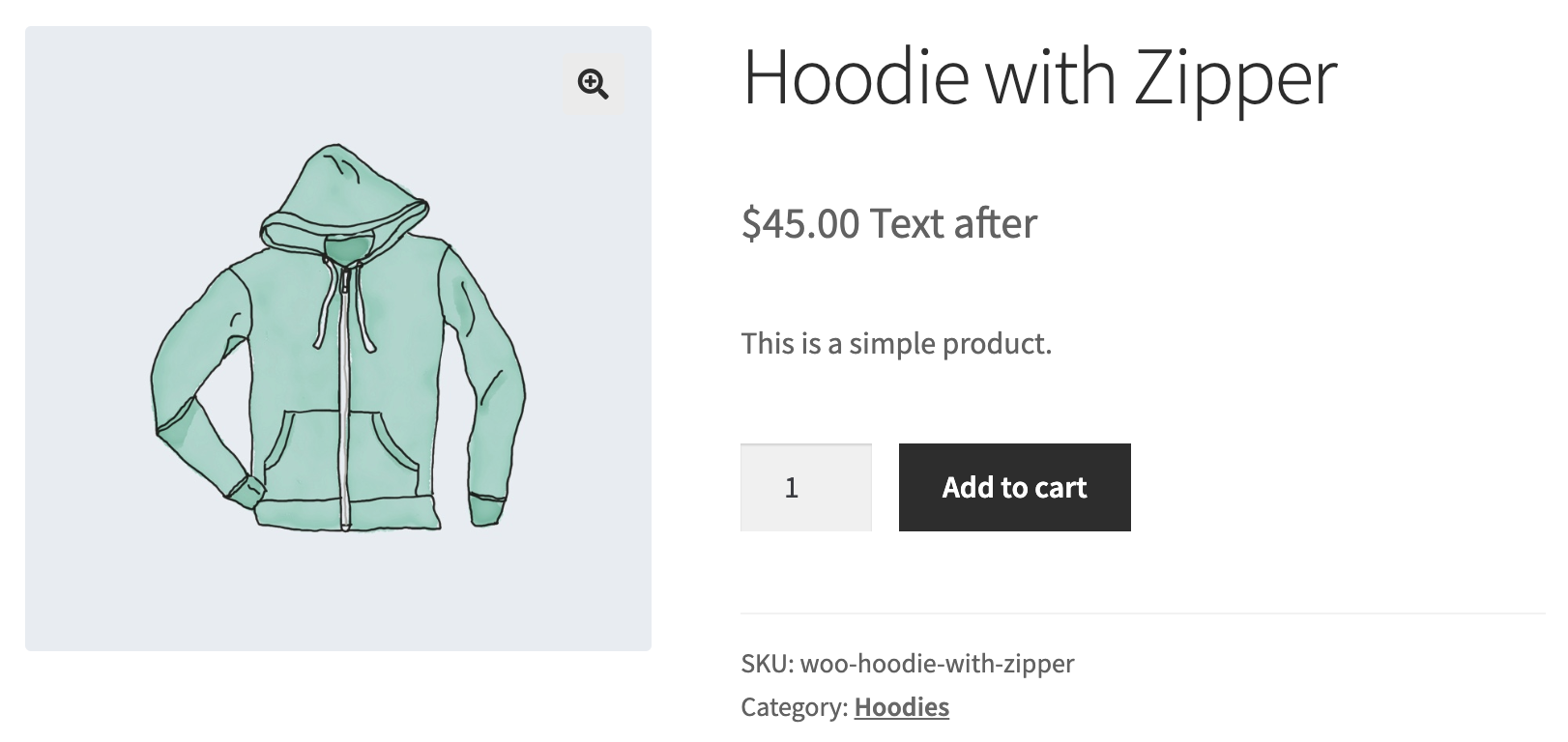
Adding text before and after the price
You can use the following code snippet to add some text before and after the price.
function yourprefix_product_price_before_after_text( $price, $product ) {
if ( !is_admin() ) { // This means it doesn't effect the price display in your dashboard, such as on the list of products
$price = 'Text before ' . $price . ' Text after';
}
return $price;
}
add_filter( 'woocommerce_get_price_html', 'yourprefix_product_price_before_after_text', 10, 2 );
Pricing will then display like this on product pages, categories, etc:
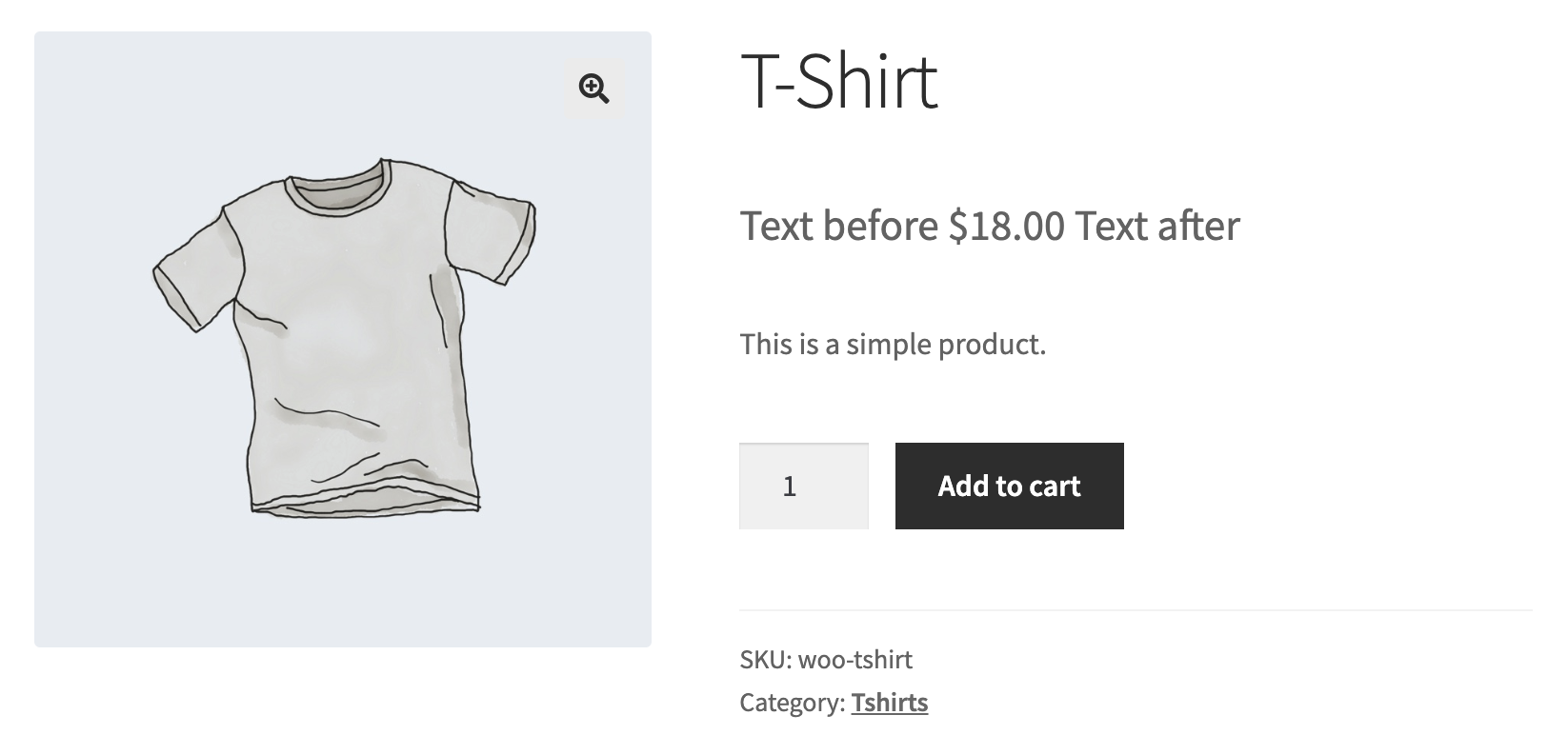
Using custom product conditions
The above examples will add text before/after the price on every product, but maybe you want to only display certain text depending on some product data, like if the product is on sale.
You can add custom product conditions based off the passed $product
object like this:
function yourprefix_product_price_before_text( $price, $product ) {
if ( !is_admin() ) { // This means it doesn't effect the price display in your dashboard, such as on the list of products
if ( $product->is_on_sale() ) {
$price = 'Text before ' . $price;
}
}
return $price;
}
add_filter( 'woocommerce_get_price_html', 'yourprefix_product_price_before_text', 10, 2 );
In the code above, I have added a condition of $product->is_on_sale()
, if this returns true, as the product is on sale, then the before text is included.
$product
is a WC_Product
object, this object has lots of getter functions that can be used to condition off the data assigned to the product.
You can review all the available functions of this object in the WooCommerce documentation.
By amending my code snippet and using the getter functions of the WC_Product
object, you can effectively add the text based on a whole host of product data conditions.
Filter hook priority
In the above examples, I am applying these filters based on a filter hook priority of 10
. While unlikely, your theme or plugins may use the same hook and be applying their own amendments to the price display, if so, you may wish to amend the hook priority accordingly.
Translation caveat
If your website is in multiple languages, you’ll want to make the before/after text a translatable string, this is more advanced and requires the loading of a textdomain. I won’t get into that here as it probably warrants an entire blog post of its own.
Looking to add an inc/exc tax suffix?
If you just want to add an including or excluding tax suffix to pricing, there is a native option to do that, which would be the recommended option for this scenario.
To use that option, if taxes are enabled for your store in WooCommerce settings, you’ll find the following option in WooCommerce > Settings > Tax > Tax options:

This option allows you to define text to show after your product prices. This could be, for example, “inc VAT” to explain your pricing. You can also have prices substituted here using one of the following: {price_including_tax}
or {price_excluding_tax}
.